vue mixin
简介
Mixins是一种让元件共用功能的方法,使用方式即是将共用功能以物件(以下称为mixin object)的方式传入mixins option。mixin object可传入元件的任何option,例如:data、computed或methods。当元件选用Mixins时,使用的mixin object会和此元件的其他option混用。
如下例,component 使用了「mixObj」和「mixObjAnother」两个mixin object。
var mixObj = {
created: function() {
this.hello();
},
methods: {
hello: function() {
console.log('Hello from Mixin');
},
},
};
var mixObjAnother = {
created: function() {
this.prompt();
},
methods: {
prompt: function() {
console.log('Prompt from Mixin');
},
hello: function() {
console.log('Hello from Mixin Another');
},
},
};
var Component = Vue.extend({
mixins: [mixObj, mixObjAnother],
});
var component = new Component();
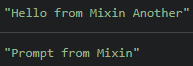
Option Merging
Hooks
当元件与使用的mixin object有相同的option时,如果是钩子(hooks),就会全部被合并为一个阵列,因此皆能被执行,并且mixin物件中的钩子会先被执行。
var mixin = {
created: function() {
console.log('mixin hook called');
},
};
new Vue({
mixins: [mixin],
created: function() {
console.log('component hook called');
},
});
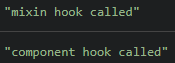
Methods, Components and Directives
如果是Methods、Components或Directives,有冲突时选用元件的option。备注:Vue.extend()
使用同样的合并策略。
var mixin = {
methods: {
foo: function() {
console.log('foo');
},
conflicting: function() {
console.log('from mixin');
},
},
};
var vm = new Vue({
mixins: [mixin],
methods: {
bar: function() {
console.log('bar');
},
conflicting: function() {
console.log('from self');
},
},
});
vm.foo();
vm.bar();
vm.conflicting();
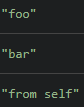
Global Mixin
全域宣告和使用Mixins。
Vue.mixin({
created: function() {
var myOption = this.$options.myOption;
if (myOption) {
console.log(myOption);
}
},
});
new Vue({
myOption: 'Hello World!',
});